Anime and Cartoon Photo Gallery from image directory in PHP
Creating an anime and cartoon photo gallery in PHP involves dynamically scanning an image directory on your server. This directory should contain anime and cartoon images in formats like JPG, PNG, or GIF. Using PHP, you'll generate thumbnails for each image found, ensuring efficient display and navigation. HTML and CSS will structure and style the gallery, potentially integrating JavaScript for features like lightboxes for full-size image viewing. Security measures such as input validation will safeguard against vulnerabilities like directory traversal attacks. The result is a responsive and interactive gallery that enhances user experience while showcasing your collection effectively.
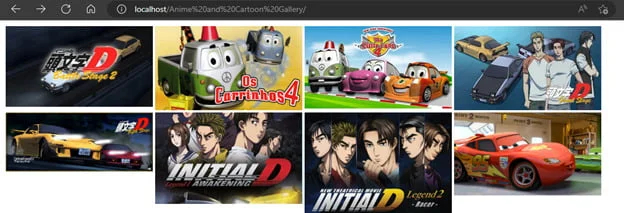
Anime and Cartoon Photo Gallery from image directory in PHP

Anime and Cartoon Photo Gallery from image directory in PHP
When you wish to make a photo gallery out of a sizable number of image files in a directory, this technique is advised.
1. Download and Include
The gallery was created using the simplelightbox jQuery library, which can be downloaded here.
Include simplelightbox.min.css and simple-lightbox.jquery.min.js.
2. HTML and PHP
The files are sourced from the images directory which also has a sub-directory thumbnail.
Specified valid image extensions in the $image_extensions Array variable and target directory in $dir.
Read every file in the directory, then establish the paths for the thumbnails and images.
Use the path in the image source if the file is a genuine image and not a directory.
For this tutorial, we use $count variable to show 4 images in a row.
Completed Code
3. CSS
4. jQuery
Initialize simpleLightbox by calling simpleLightbox() method on <a> of gallery class.
5. Final Notes
Only the target folder where the photos are stored needs to be specified; if any other files are located there instead of the image, the PHP script bypasses them.
You can change the layout and use any other jQuery library for the photo gallery.
What's Your Reaction?
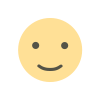
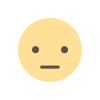
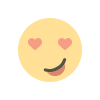
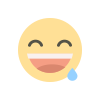
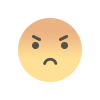
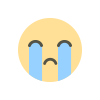
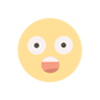